How does ReactJS work? (Part 1)
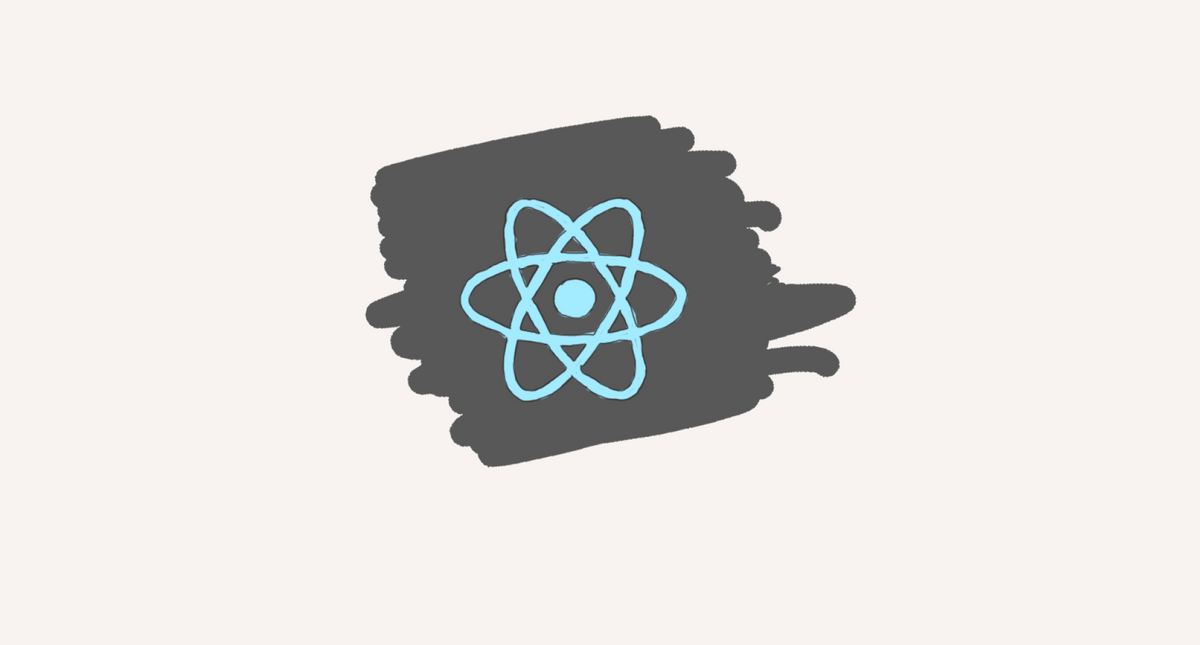
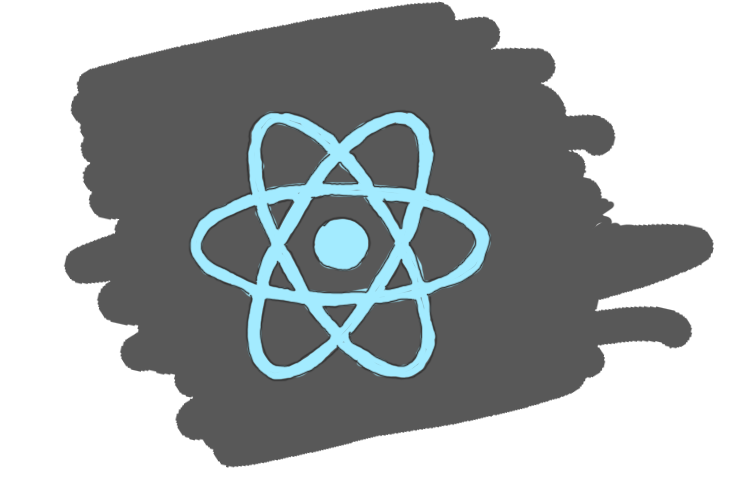
I've been working as an independent developer for some time now, and it doesn't take long for one to notice that most jobs are done through ReactJS.
For the uninitiated, ReactJS is a specific way to code Javascript. It's called a framework, and there are dozens out there.
None as popular as React, though. So, this blog is meant for me to brush up on the basics.
It'll need a couple of parts, I think three. So bear with me.
What is the difference between ReactJS and plain JS?
To get into that, it's important to first understand how most websites are built.
I drew a poor rendition of Twitter below, but it should be able to serve our needs.
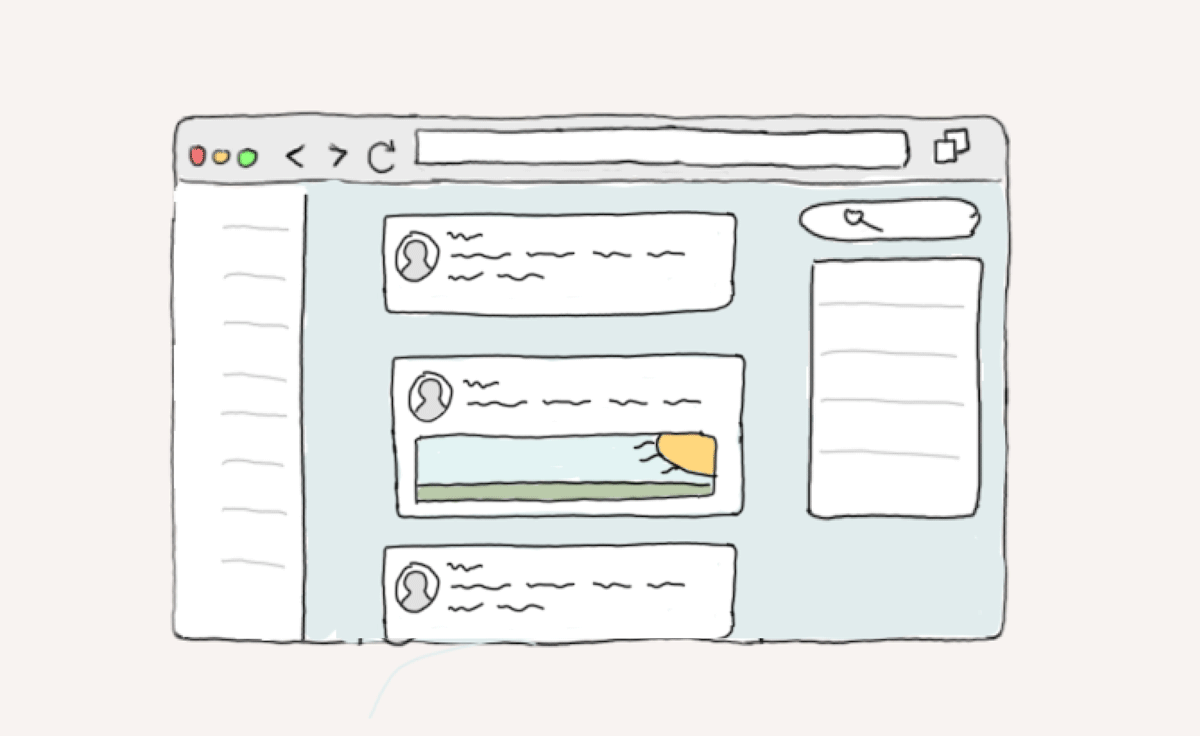
When you look at Twitter's home page, you'll notice the tweets, the left-hand side menu, etc.
I think most people know by now how websites are built, but basically they're built on three main tenets:
- HTML: you choose a bunch of elements to display on a screen (tweets, search bar, etc)
- CSS: you decide what your elements look like (all tweets will have a white background)
- Javascript: you do everything else with Javascript (when you click on a tweet, you will go to a new page)
It was convention to separate projects by file types, so for a while, projects looked like someone having a bunch of HTML files, a bunch of CSS styles, and a bunch of Javascript files.
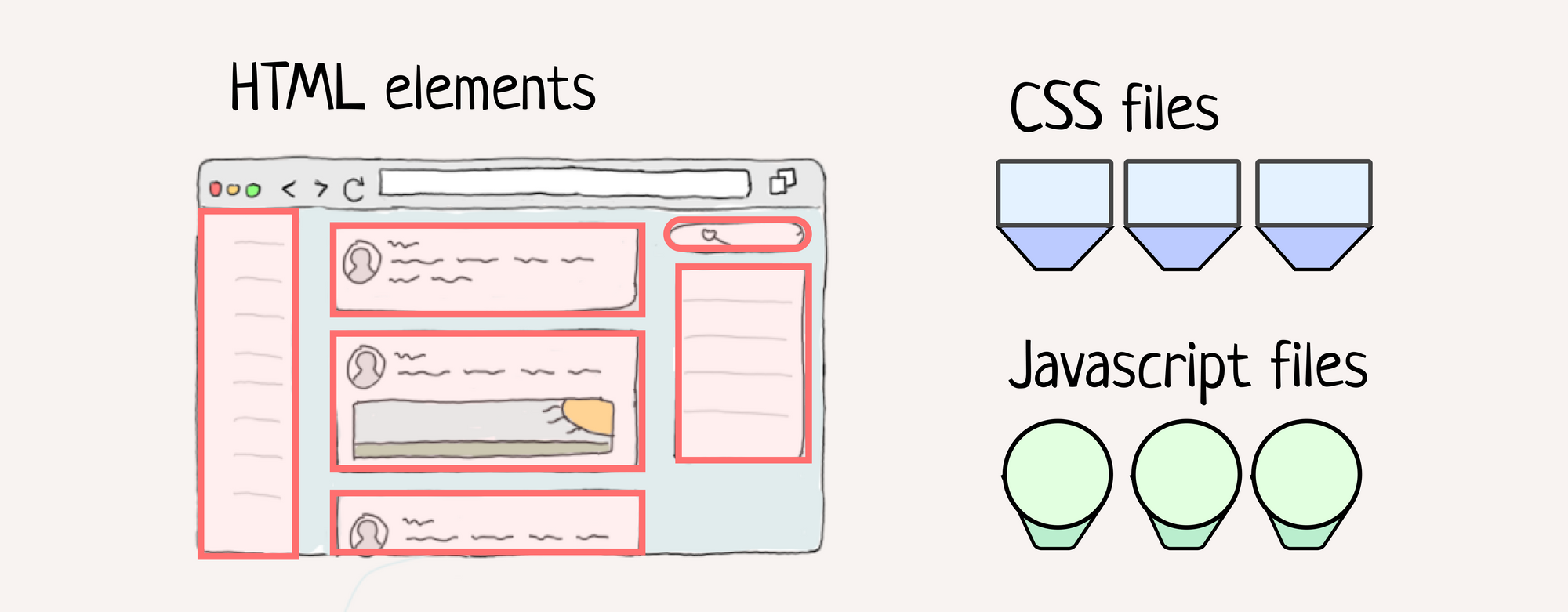
Over time, software developers started to organize their code differently
Now, developers are some smart people.
After creating projects like this for a while, the developer community realized that code became a lot easier to work with once it was organized by context.
What does that mean? Basically, it became convention to think about code as HTML elements having dedicated CSS and JS files.
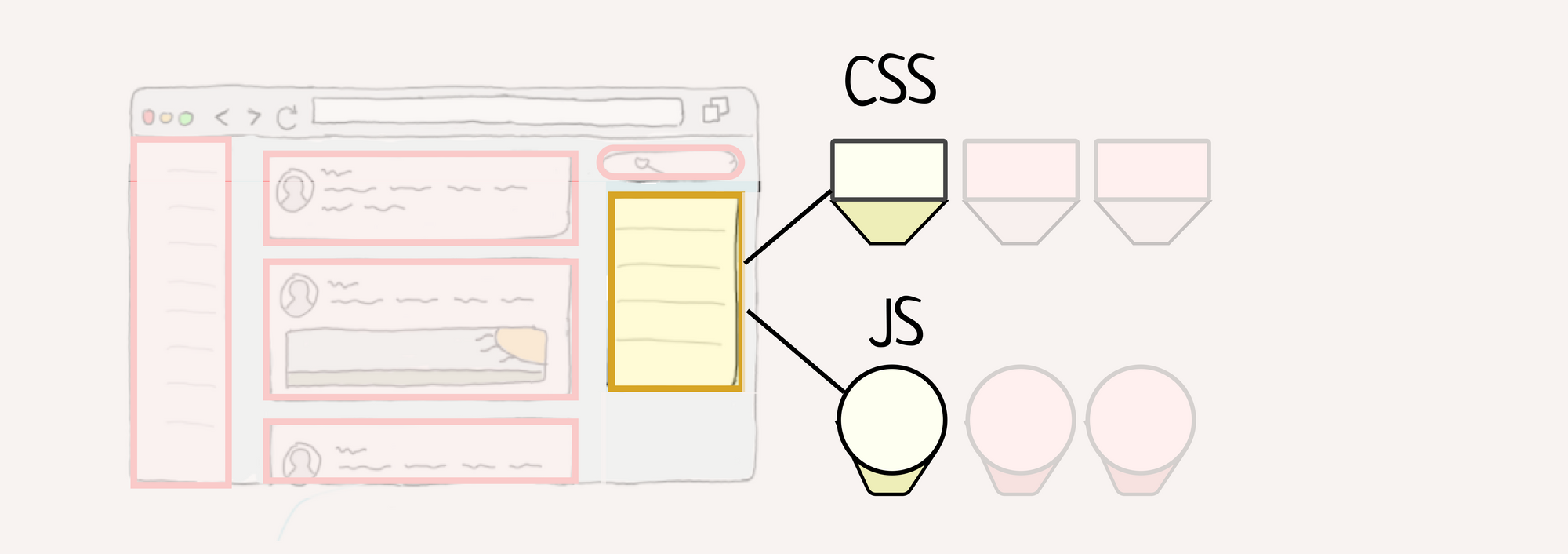
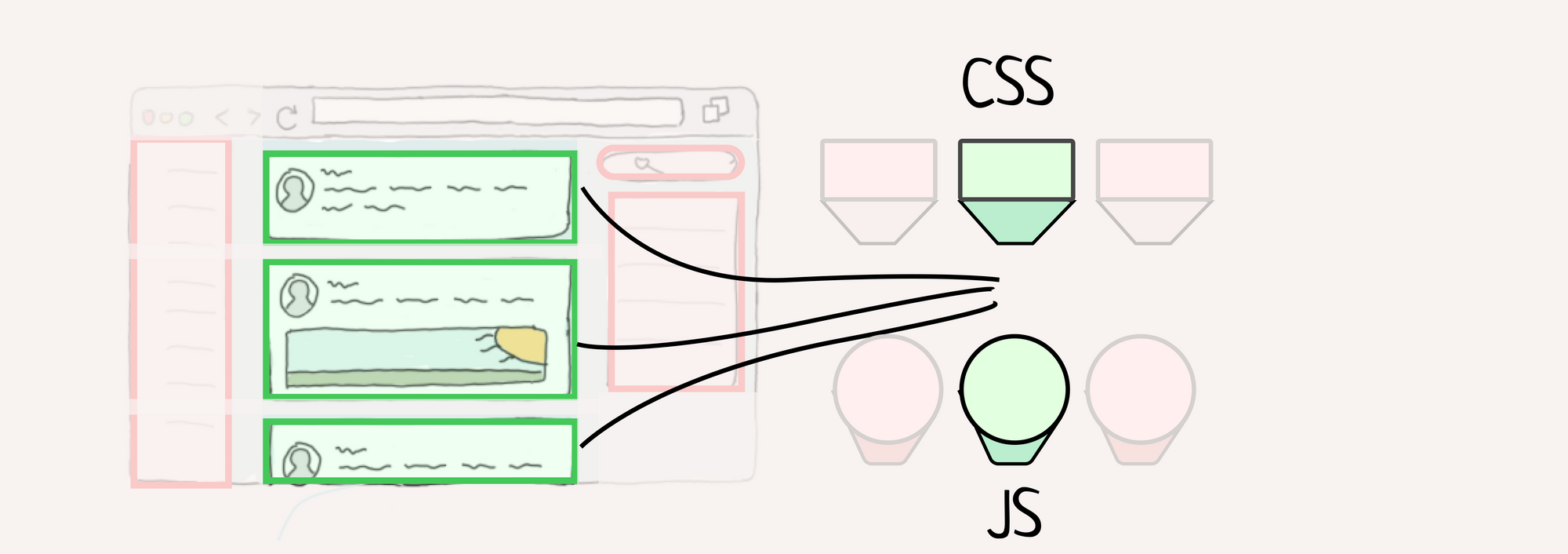
When something needs to change, there are only a few files that should be affected, and everything else should work independently from that code.
This is essentially how I think about components.
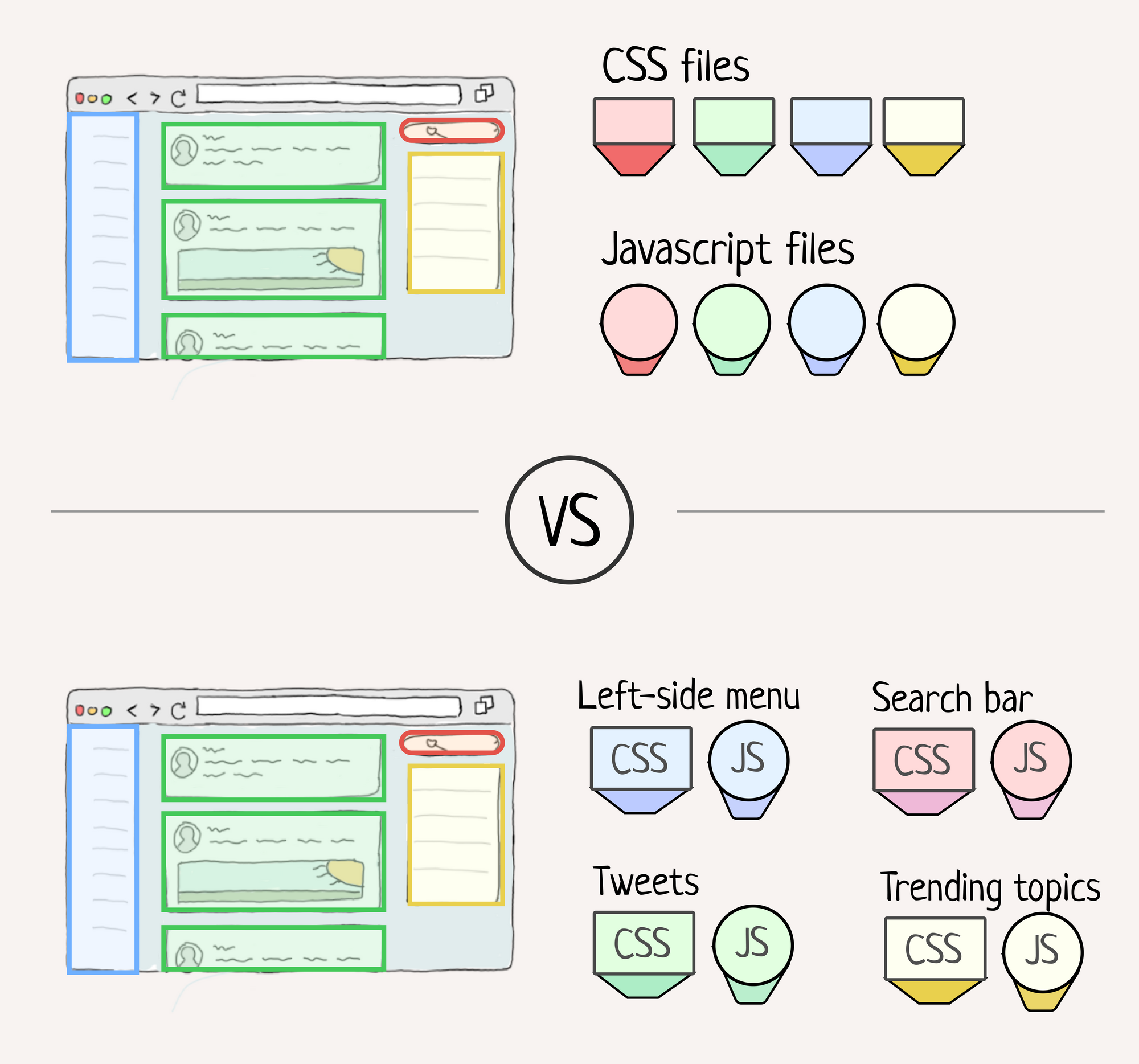
A component is supposed to be reusable and self-contained
Once I understand that every website is essentially a bunch of components stacked together, it became easier for me to see it out in the wild.
Let's take a look at a tweet again. But let's draw it in 3D space.
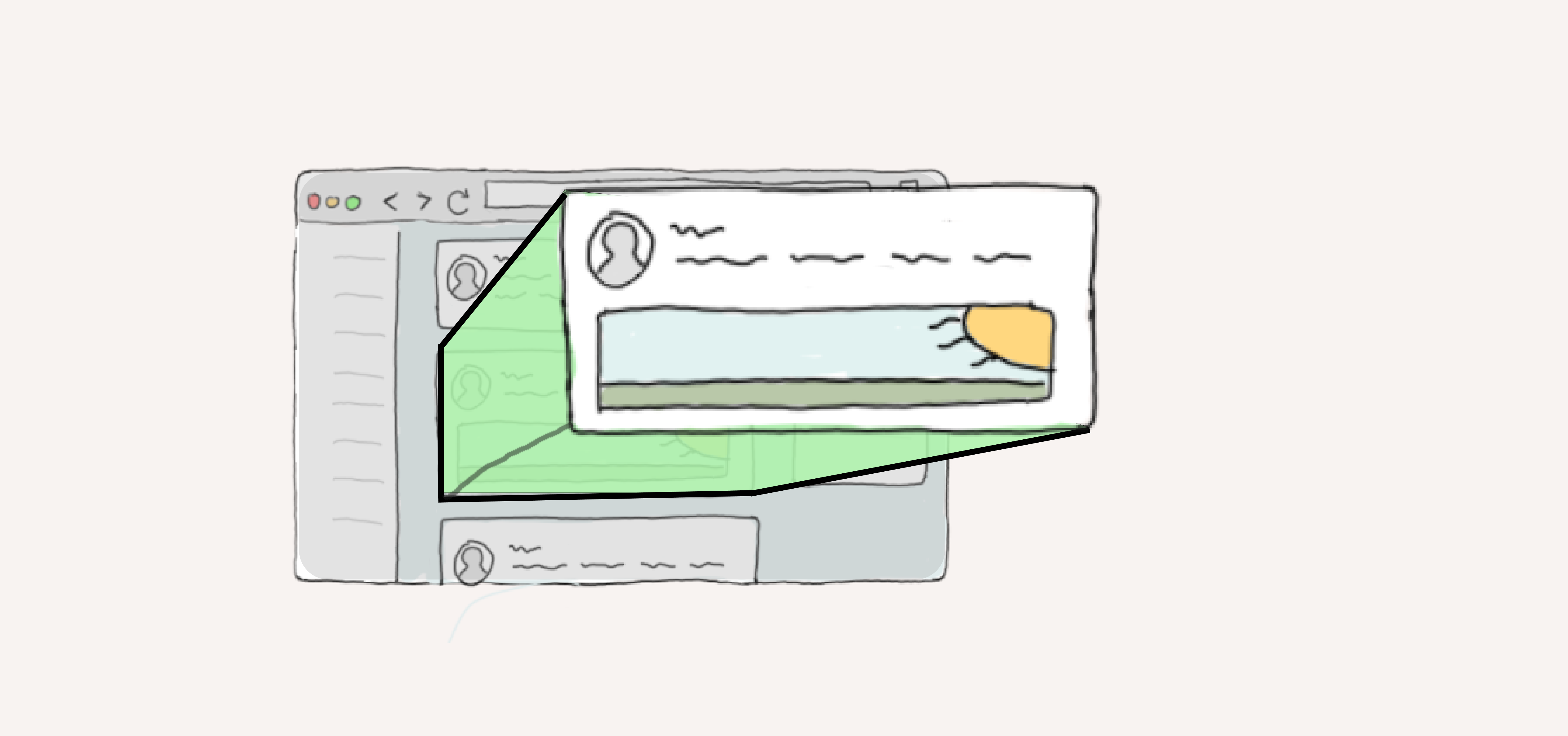
If we were to break down the Tweet into its parts, we would see a
- profile picture of some sort
- some text
- and an image.
It would be safe of us to assume, then, that these things can also be broken down into components.
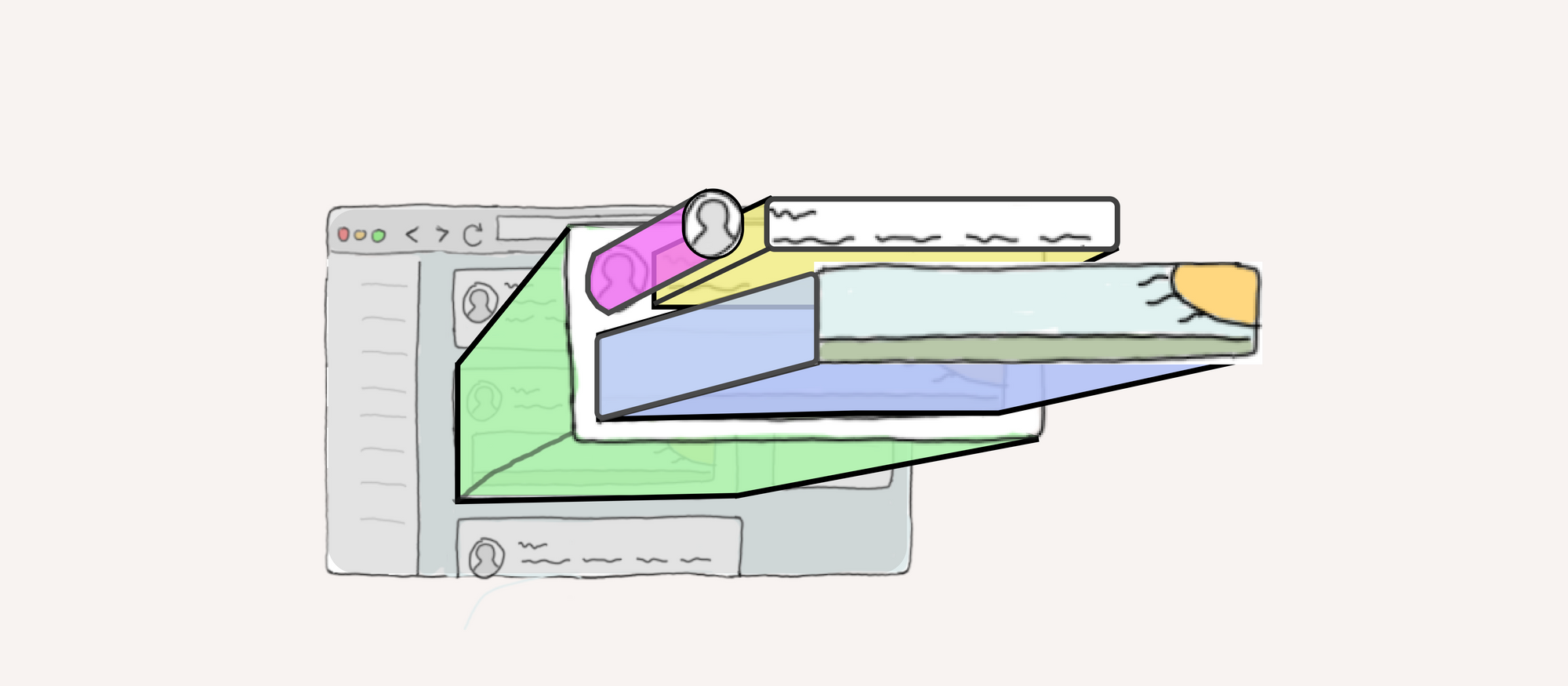
We just broke down the Tweet into its parts.
And this is the React way; the smaller the pieces, the more accessible it is to think about it.
Because components can live inside one another, they start to develop a tree
This is not only true for React, but all of HTML. One piece of code lives inside another piece of code, and that piece of code in turn lives inside another.
If we flatten our 3D structure a bit, you start to see an interesting thing happen: the result looks like a tree.
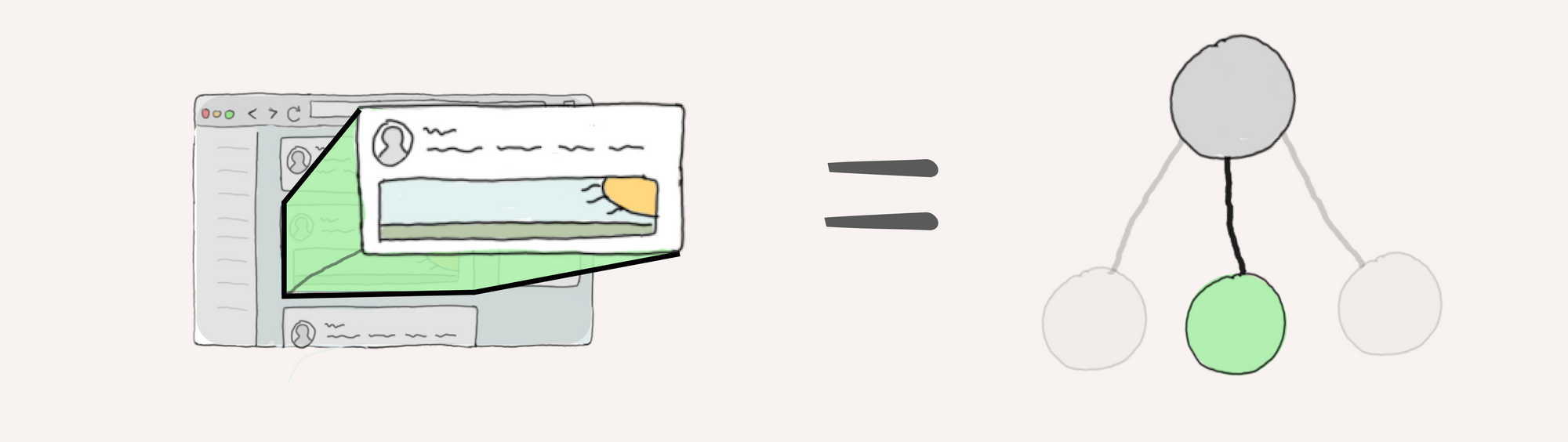
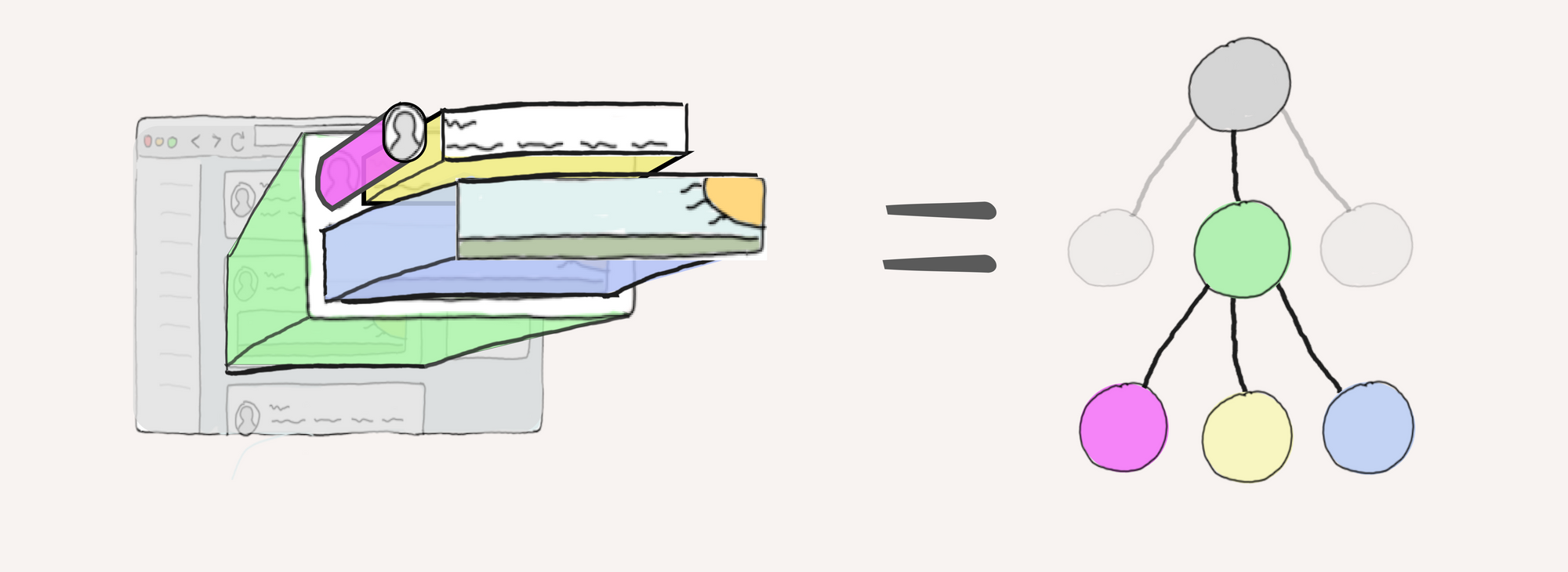
We call this tree thing a DOM.
It's basically the skeleton of a webpage: it shows all the components of a website at a given time, and where exactly those components live.
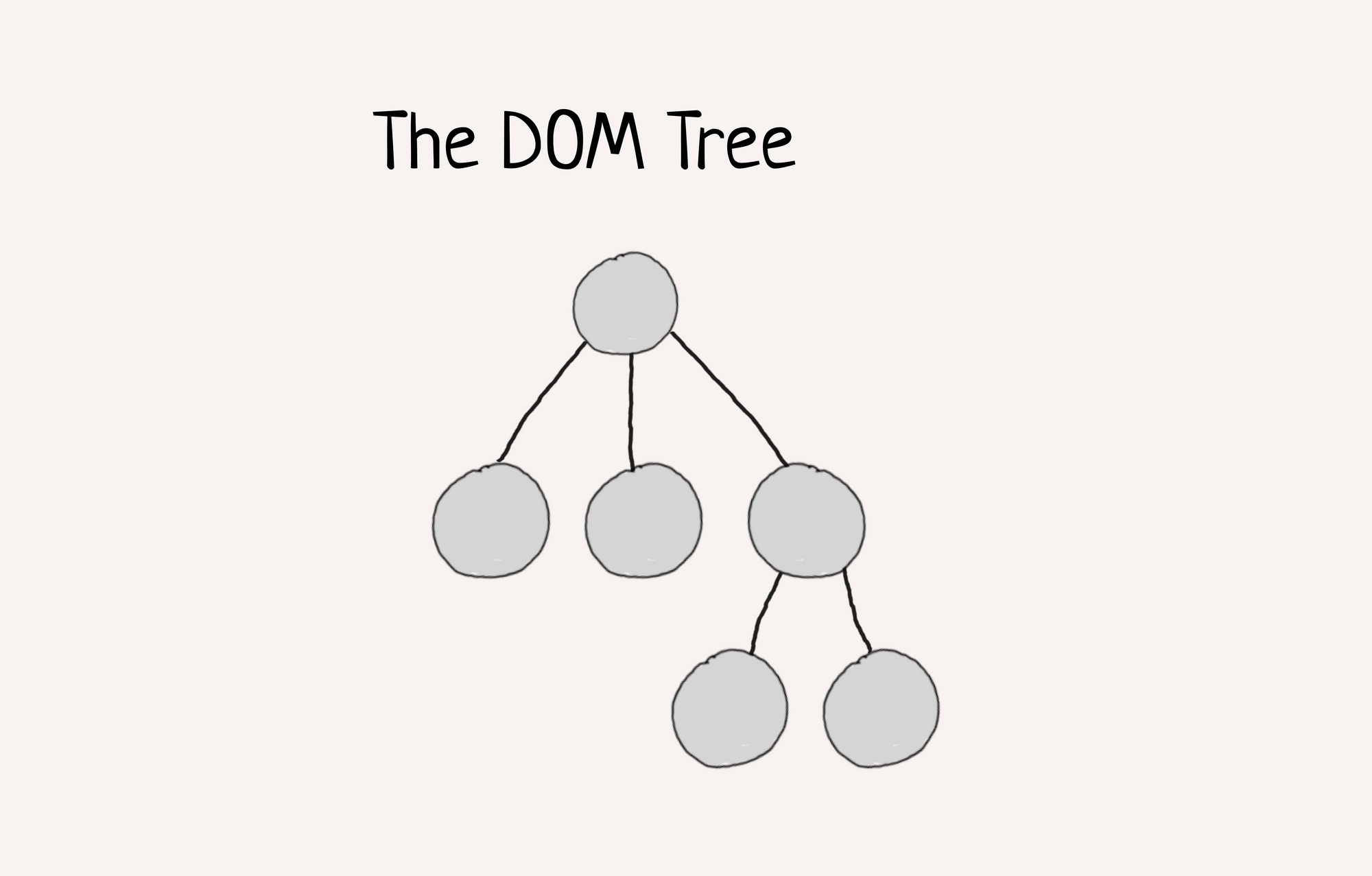
The main point of React is that it created a virtual DOM
All of my discussion has been leading to this point: React's virtual DOM.
The basic idea is that React:
- React makes a copy of the DOM and calls it the virtual DOM
- in the virtual DOM, developers can do things better and faster than regular Javascript because things take longer in the DOM than in the Virtual DOM
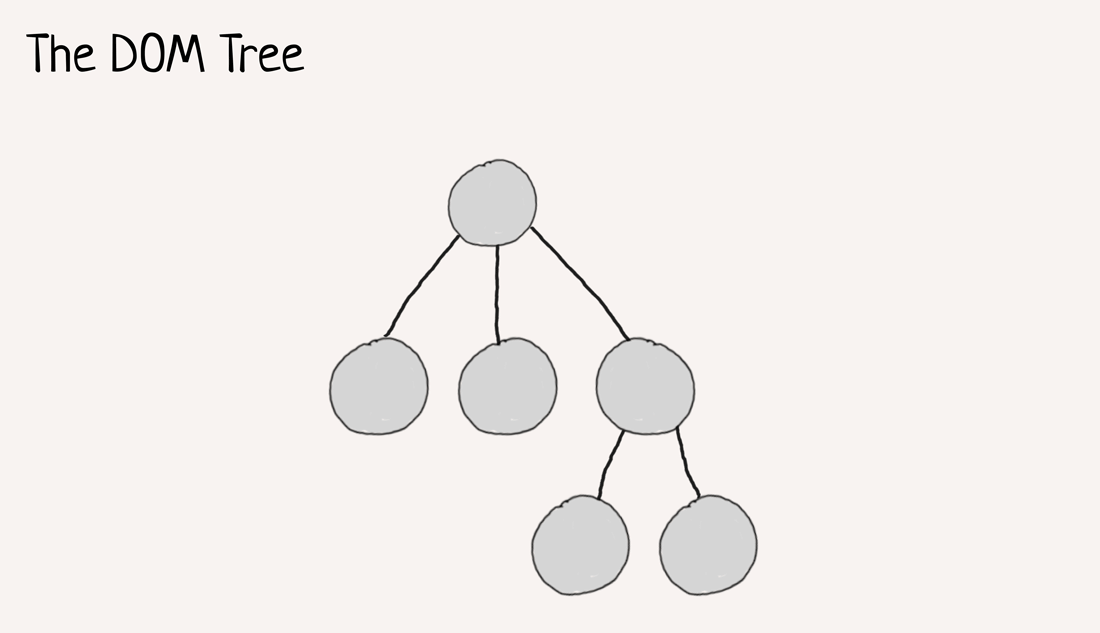
Now, that we're in the virtual DOM, we can do things a lot quicker. React abstracted a bunch of these concepts, so things like changing the color of a component or removing it entirely can be done with the super powerful Javascript.
- the virtual DOM changes to reflect the logic
- the virtual DOM then tells the real DOM what changed
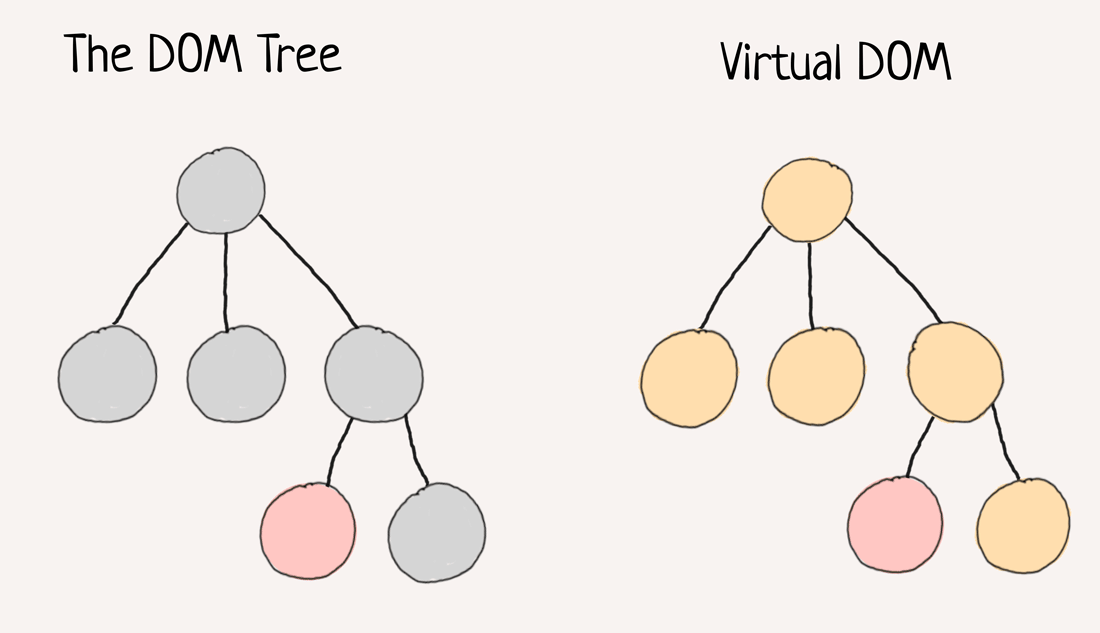
And this is the magic of React. The react virtual DOM makes it easier for the computer to change one part of a website without changing the rest.
I mentioned this is only part of how React works. In the next blog I write, I'll take a look at how we pass information between these components, and why it is important.